Where to get ASIHTTPRequest:
- Github project page: http://github.com/pokeb/asi-http-request/tree
- Download the latest version: http://github.com/pokeb/asi-http-request/tarball/master
- License (BSD): http://github.com/pokeb/asi-http-request/tree/master/LICENSE
- Google Group: http://groups.google.com/group/asihttprequest
- Lighthouse bug base: http://allseeing-i.lighthouseapp.com/projects/27881/home
What is ASIHTTPRequest?
ASIHTTPRequest is an easy to use wrapper around the CFNetwork API that makes some of the more tedious aspects of communicating with web servers easier. It is written in Objective-C and works in both Mac OS X and iPhone applications.
It is suitable performing basic HTTP requests and interacting with REST-based services (GET / POST / PUT / DELETE). The included ASIFormDataRequest subclass makes it easy to submit POST data and files using multipart/form-data.
Features
- A straightforward interface for submitting data to and fetching data from webservers
- Download data to memory or directly to a file on disk
- The ability to submit files on local drives as part of POST data, compatible with the HTML file input mechanism
- Easy access to request and response HTTP headers
- Progress delegates (NSProgressIndicators and UIProgressViews) to show information about download AND upload progress
- Auto-magic management of upload and download progress indicators for operation queues
- Basic, Digest and NTLM authentication support, credentials are automatically for the duration of a session, and can be stored for later in the Keychain.
- Cookie support
- NEW! Requests can continue to run when your app moves to the background (iOS 4+)
- GZIP support for response data AND request bodies
- The included ASIDownloadCache class lets requests transparently cache responses, and allow requests for cached data to succeed even when there is no network available!
- NEW! ASIWebPageRequest - download complete webpages, including external resources like images and stylesheets. Pages of any size can be indefinitely cached, and displayed in a UIWebview / WebView even when you have no network connection.
- Easy to use support for Amazon S3 - no need to fiddle around signing requests yourself!
- Full support for Rackspace Cloud Files, contributed by Mike Mayo of Rackspace.
- NEW! Client certificates support
- Supports manual and auto-detected proxies, authenticating proxies, and PAC file auto-configuration. The built-in login dialog lets your iPhone application work transparently with authenticating proxies without any additional effort.
- Bandwidth throttling support
- Support for persistent connections
- Supports synchronous & asynchronous requests
- Get notifications about changes in your request state via delegation or NEW! blocks (Mac OS X 10.6, iOS 4 and above)
- Comes with a broad range of unit tests
ASIHTTPRequest comes with a example applications for Mac and iPhone that demonstrate some of the features.
ASIHTTPRequest is partly based on code from Apple’s ImageClient code samples, so if it doesn’t meet your needs, take a look at their CFNetwork examples for more.
ASIHTTPRequest is compatible with Mac OS 10.5 or later, and iOS 3.0 or later.
An overview of the classes
Main classes
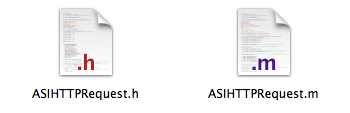
ASIHTTPRequest
Handles the basics of communicating with webservers, including downloading and uploading data, authentication, cookies and progress tracking.
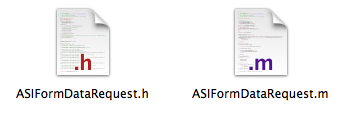
ASIFormDataRequest
A subclass of ASIHTTPRequest that handles x-www-form-urlencoded and multipart/form-data posts. It makes POSTing data and files easy, but you do not need to add this to your project if you want to manage POST data yourself or don’t need to POST data at all.
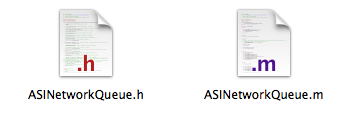
ASINetworkQueue
A subclass of NSOperationQueue that may be used to track progress across multiple requests. You don’t need this if you only need to perform one request at a time, or prefer to track the progress of each request individually.
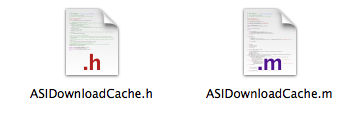
ASIDownloadCache
This class allows ASIHTTPRequest to transparently cache responses from webservers. Requests can be configured to use cached content when remote data has not been updated since it was last downloaded, when the network is available, or whenever cached data is available.
If you do not wish to use caching, or have written your own cache, you do not need to include this class.
Support classes
You will not generally need to use these classes directly, they are used behind the scenes by the main classes above.
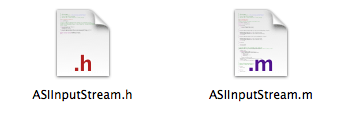
ASIInputStream
A helper class used by ASIHTTPRequest when uploading data. You must include this class in your project to use ASIHTTPRequest.
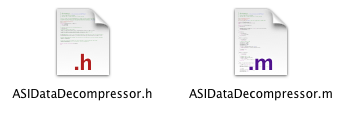
ASIDataDecompressor
A helper class used by ASIHTTPRequest to inflate (decompress) gzipped content. You must include this class in your project to use ASIHTTPRequest.
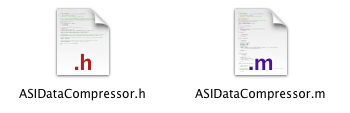
ASIDataCompressor
A helper class used by ASIHTTPRequest to deflate (compress) content. You must include this class in your project to use ASIHTTPRequest.
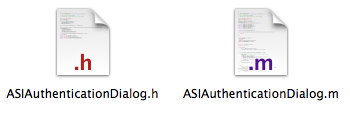
ASIAuthenticationDialog
This class allows ASIHTTPRequest to present a login dialog when connecting to webservers that require authentication, and authenticating proxies. It is required in all projects targeting iPhone OS, though not for Mac OS projects.
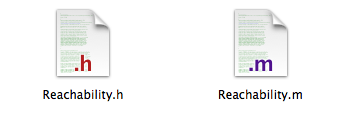
Reachability
This class was written by Andrew Donoho as a drop-in replacement for Apple’s Reachability class. It allows ASIHTTPRequest to be notified when the network connection changes from WWAN to WiFi, or vice-versa. You must include this class in iPhone projects, but not in Mac projects.
You may find this class useful in detecting the status of network availability in your own applications - find out more.
Protocols and configuration
You must include all these files in your project.
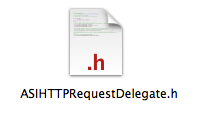
ASIHTTPRequestDelegate
This protocol specifies the method that a delegate of an ASIHTTPRequest may implement. All these methods are optional.
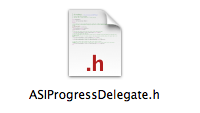
ASIProgressDelegate
This protocol lists the methods that an uploadProgressDelegate or downloadProgressDelegate may implement. All these methods are optional.
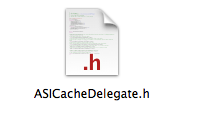
ASICacheDelegate
This protocol is used to specify the methods that a download cache must implement. If you want to write your own download cache, make sure it implements the required methods in this protocol.
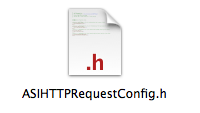
ASIHTTPRequestConfig.h
This file defines global configuration options that are set at compile time. Use the options in this file to turn on various debugging options that print information about what a request is doing to the console. Don't forget to turn these off in shipping applications!